Integrating AI and Machine Learning (ML) into a Laravel application can enhance its capabilities by providing advanced features such as predictive analytics, natural language processing, and personalized recommendations. Here are some steps and considerations for integrating AI and ML into a Laravel application:
1. Determine Use Cases
Identify the specific use cases for integrating AI/ML in your Laravel application. Common use cases include:
Predictive Analytics: Forecasting future trends or behaviors.
-
Natural Language Processing (NLP): Understanding and generating human language.
-
Recommendation Systems: Suggesting products, content, or actions.
-
Image and Video Analysis: Processing and analyzing visual data.
-
Automated Customer Support: Chatbots and virtual assistants.
2. Choose the Right Tools and Libraries
Select appropriate AI/ML libraries and tools based on your use cases. Popular choices include:
Python Libraries: TensorFlow, Keras, PyTorch, Scikit-learn.
-
PHP Libraries: PHP-ML, Rubix ML.
-
APIs and Services: Google Cloud AI, AWS SageMaker, IBM Watson, Azure Cognitive Services.
3. Setup Laravel Project
Ensure your Laravel project is set up and ready for integration. You can create a new Laravel project or use an existing one.
composer create-project –prefer-dist laravel/laravel your_project_name
4. Integrate AI/ML Models
Using PHP Libraries
Install PHP-ML or Rubix ML: Add the required library to your Laravel project using Composer.composer require php-ai/php-ml
composer require rubix/ml
Develop and Use Models: Create and use ML models directly within your Laravel application.
use Phpml\Classification\KNearestNeighbors;
use Phpml\ModelManager;
// Example: Train and save a model
$classifier = new KNearestNeighbors();
$classifier->train($samples, $labels);
$modelManager = new ModelManager();
$modelManager->saveToFile($classifier, ‘path_to_model_file’);
// Example: Load and predict with a model
$classifier = $modelManager->restoreFromFile(‘path_to_model_file’);
$predictions = $classifier->predict($samples);
5. Data Preparation and Processing
Prepare and preprocess your data before feeding it into your AI/ML models. This can involve cleaning, normalizing, and transforming data. Use Laravel’s Eloquent ORM or query builder to manage your data.
$data = DB::table(‘your_table’)->get();
$processedData = $data->map(function($item) {
// Perform necessary data transformations
return [
‘feature1’ => $item->feature1,
‘feature2’ => $item->feature2,
// …
];
});
6. Implement AI/ML Features in Laravel
Integrate the AI/ML functionality into your application’s features, such as:
-
Routes and Controllers: Define routes and create controllers to handle AI/ML-related requests.
-
Views: Display AI/ML predictions or recommendations in your views.
-
Jobs and Queues: Use Laravel’s job and queue system to handle AI/ML tasks asynchronously.
7. Testing and Validation
Thoroughly test your AI/ML integration to ensure it works as expected. Validate model predictions and performance.
8. Monitor and Maintain
Continuously monitor and maintain your AI/ML models and integration. Update models as needed and ensure the system scales with increased usage.
app/
Http/
Controllers/
AiController.php
Services/
AiService.php
config/
ai.php
routes/
web.php
resources/
views/
ai.blade.php
Conclusion
Integrating AI and ML into a Laravel application involves choosing the right tools, developing and deploying models, and embedding them into your application logic. With careful planning and execution, you can enhance your Laravel application with powerful AI/ML capabilities.
Unlock the potential of Laravel with XcelTec: Seamlessly integrate AI & Machine Learning for smarter, more efficient solutions.
Visit our website: https://www.xceltec.com/
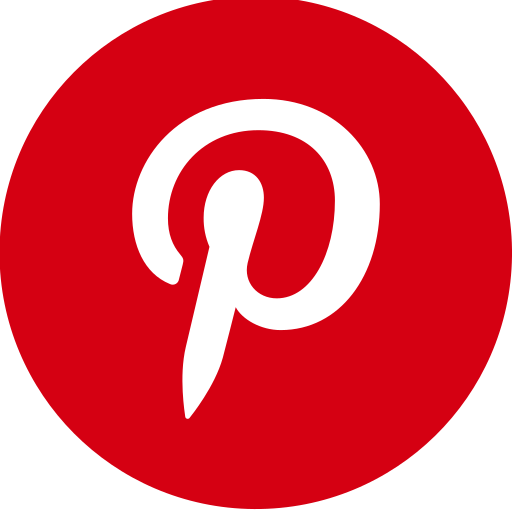