Flutter provides a fast and flexible way to build cross-platform applications. However, performance issues can arise if apps do not follow best practices. Users expect smooth animations, fast loading times, and minimal lag. Ignoring performance can lead to slow rendering, high memory usage, and frequent crashes.
This guide covers Flutter app performance optimization techniques that help developers build faster applications. It includes best practices for Flutter app speed enhancement, techniques to boost Flutter app performance, and Flutter app optimization strategies that prevent slowdowns.
Understanding Flutter App Performance
Flutter uses a single codebase to create apps for multiple platforms. The framework relies on the Skia Graphics Engine, which renders UI efficiently. However, poor coding practices can affect rendering speed and memory usage.
Two main execution methods impact performance:
1. JIT (Just-In-Time) Compilation: Speeds up development by compiling code dynamically. However, it affects performance in production.
2. AOT (Ahead-Of-Time) Compilation: Pre-compiles the app, resulting in faster execution.
Understanding how Flutter rebuilds widgets and manages UI interactions helps in applying Flutter app optimization strategies effectively.
Why Optimize Flutter App Performance?
Optimizing Flutter app performance can result in several benefits, including:
- Improved User Experience: Responsive apps keep users satisfied and engaged.
- Increased Retention Rates: Faster apps encourage users to return and explore more features.
- Better App Ratings: High performance often translates into better reviews and higher rankings in app stores.
Techniques to Boost Flutter App Performance
1. Efficient Widget Management
Flutter apps rely on widgets. Poor widget management can lead to unnecessary rebuilds, affecting speed.
- Use const constructors whenever possible to reduce widget rebuilds.
- Avoid unnecessary widget tree nesting, which increases rendering time.
- Use StatelessWidget instead of StatefulWidget when data does not change.
- Implement ValueKey to retain widget states efficiently.
- Choose State Management Solutions like Provider, Riverpod, or Bloc to control unnecessary re-renders.
Applying these Flutter app performance optimization techniques ensures better resource management and a smooth UI.
2. Reducing Rendering Overhead
Flutter redraws UI elements frequently. Reducing overdraw and optimizing how widgets refresh can prevent lag.
- Use RepaintBoundary to isolate widgets that do not need frequent updates.
- Replace heavy ListView or GridView with ListView.builder to load items only when required.
- Avoid using large images that slow down rendering.
- Use SizedBox instead of Container when only width and height are needed.
- Reduce Opacity widget usage and prefer transparent PNGs instead.
These best practices for Flutter app development speed enhancement reduce rendering workload and keep UI interactions smooth.
3. Improving App Load Time
Users expect apps to load instantly. Long loading times lead to frustration and higher bounce rates.
- Apply lazy loading techniques to load content only when needed.
- Optimize assets by using compressed images and vector graphics instead of large PNGs.
- Reduce app size by removing unused dependencies.
- Implement code splitting to load features on demand instead of all at once.
- Use cached_network_image to store frequently used images locally.
By following these Flutter app performance optimization techniques, developers can prevent delays in app launch and content loading.
4. Memory and Resource Management
High memory usage causes slow performance and frequent crashes. Managing resources efficiently ensures the app runs without consuming unnecessary system resources.
- Dispose of controllers properly, including TextEditingController and AnimationController.
- Use Flutter DevTools to track memory leaks.
- Limit object allocations to reduce garbage collection overhead.
- Use image caching strategies to prevent redundant memory usage.
- Avoid large lists in memory; use pagination to load only required data.
Applying these Flutter app optimization strategies prevents memory-related issues and enhances responsiveness.
5. Managing Animations and Transitions
Flutter supports smooth animations, but inefficient handling can impact performance.
- Keep animations under 16ms per frame to maintain a smooth 60fps experience.
- Use TickerMode to disable animations when not visible.
- Use AnimatedBuilder instead of setState to avoid unnecessary widget rebuilds.
- Prefer Lottie animations over heavy GIFs.
These techniques contribute to a better user experience by maintaining fluid transitions without frame drops.
6. Optimizing API Calls and Network Requests
Fetching and displaying data efficiently prevents slow response times.
- Use Dio or HTTP package for network requests with error handling.
- Apply caching techniques to avoid redundant API calls.
- Implement pagination instead of fetching large datasets.
- Optimize JSON parsing with json_serializable instead of default decode methods.
- Use WebSockets for real-time data updates instead of frequent API polling.
These techniques to boost Flutter app performance improve data loading speed while reducing unnecessary network activity.
Tools for Monitoring Performance
Flutter provides tools to analyze and fix performance bottlenecks.
- Flutter DevTools – Helps track UI rendering speed, memory usage, and network requests.
- Dart Observatory – Analyzes execution time and detects slowdowns.
- Firebase Performance Monitoring – Measures app response time in real-world conditions.
- Sentry for Flutter – Identifies crashes and performance issues.
Regularly using these tools helps in applying best practices for Flutter app speed enhancement efficiently.
Best Practices for Long-Term Performance Maintenance
Maintaining performance requires continuous monitoring and updates.
- Keep dependencies updated to benefit from Flutter improvements.
- Run profiling tests regularly using Flutter Driver or Integration Testing.
- Reduce unused libraries and third-party packages to decrease app size.
- Refactor code to remove redundant computations and improve maintainability.
- Test across different devices to detect platform-specific issues.
By following these Flutter app optimization strategies, developers can maintain app efficiency in the long run.
Also read: Top 20 Flutter Apps in 2025: A Sneak Peek at Cross-Platform Brilliance
Conclusion
Building a high-performance Flutter app requires careful management of widgets, rendering efficiency, memory usage, and network optimization. By following Flutter app performance optimization techniques, developers can improve responsiveness, reduce lag, and deliver a smoother experience. Applying best practices for Flutter app speed enhancement ensures faster loading times and better resource handling.
For businesses looking for affordable Flutter app development services and solutions, Shiv Technolabs offers expert guidance and tailored solutions. Whether it’s performance tuning, feature development, or end-to-end Flutter app development, their team ensures quality and efficiency. By partnering with professionals, businesses can build apps that not only perform well but also provide an outstanding user experience.
Want to optimize your Flutter app further? Get in touch with Shiv Technolabs for customized solutions that fit your project’s needs.
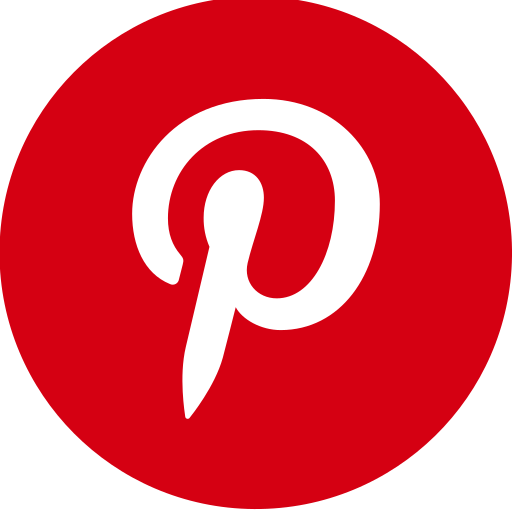